I needed a Sitecore Form with a country dropdown list. It would also have to default to the country that Sitecore’s Geo IP would detect. To accomplish this I had to do the following steps.
Create the class
The class inherits from the DropDownListView Model and implements the following code. The list of the countryselectoritems is what the view (later step) will use to populate the dropdown list.
This is the what the countryselectoritem is defined as:
public class CountrySelectorItem
{
public string CountryCode { get; set; }
public string CountryName { get; set; }
public string SelectedItem { get; set; }
}
The code is as follows:
using Sitecore.Diagnostics;
using Sitecore.Data.Items;
using Sitecore.ExperienceForms.Mvc.Models.Fields;
using System.Collections.Generic;
using static Supernatural.Feature.WhitePaper.Models.CountryDropDownModel;
namespace Supernatural.Feature.WhitePaper.FormFields
{
public class CountryDropDown : DropDownListViewModel
{
protected override void InitItemProperties(Item item)
{
Assert.ArgumentNotNull((object)item, nameof(item));
base.InitItemProperties(item);
}
protected override void UpdateItemFields(Item item)
{
Assert.ArgumentNotNull((object)item, nameof(item));
base.UpdateItemFields(item);
}
public List<CountrySelectorItem> Countries()
{
List<CountrySelectorItem> countrylist = FormHelper.GetCountryList();
return countrylist;
}
}
}
The function FormHelper.GetCountryList() can be implemented any way you want. I am using Sitecore XP so there is a country list available. I simply build a list of CountrySelectorItems. One of the important parts of the code is the Geo IP country retrieval. We will retrieve the country from the IP address using Sitecore’s Geo IP lookup and set the SelectedItem to that country. That way when we render the dropdown we know which country value should be selected (aka defaulted). Here is one example:
public static List<CountrySelectorItem> GetCountryList()
{
List<CountrySelectorItem> countrylist = new List<CountrySelectorItem>();
Database contextDB;
if (Sitecore.Context.Database.Name == "core")
{
contextDB = Sitecore.Configuration.Factory.GetDatabase("master");
}
else
{
contextDB = Sitecore.Context.Database;
}
Item countriesfolder = contextDB.GetItem(LanguageCountry.CountriesFolder);
string activecountrycode = GeoIpHelper.GetGeoIpCountry();
foreach (Item country in countriesfolder.GetChildren().Where(a => a.DoesItemInheritFrom(LanguageCountry.CountryTemplate)))
{
string countrycode = country.Fields[LanguageCountry.Fields.Country].Value;
CountrySelectorItem countrySelectorItem = new CountrySelectorItem();
countrySelectorItem.CountryName = country.DisplayName;
countrySelectorItem.CountryCode = countrycode;
if (!string.IsNullOrEmpty(activecountrycode) && countrySelectorItem.CountryCode.ToUpper() == activecountrycode.ToUpper())
{
countrySelectorItem.SelectedItem = countrySelectorItem.CountryCode;
}
countrylist.Add(countrySelectorItem);
}
return countrylist;
}
Create the View
The view is simple. I took an existing view from a list rendering and used that as a starting point.
@using Sitecore.ExperienceForms.Mvc.Html
@using Supernatural.Feature.Media.Models
@model Supernatural.Feature.Mmedia.FormFields.CountryDropDown
@{
var countrylist = Model.Countries();
}
@Html.DisplayTextFor(t => Model.Title)
Select Your Country @if (countrylist != null) {
foreach (CountryDropDownModel.CountrySelectorItem countryselitem in countrylist)
{
if (countryselitem.SelectedItem == countryselitem.CountryCode)
{
<option class="flags-@countryselitem.CountryCode.ToLower()" selected value="@countryselitem.CountryCode">@countryselitem.CountryName</option>
}
else
{
<option class="flags-@countryselitem.CountryCode.ToLower()" value="@countryselitem.CountryCode">@countryselitem.CountryName</option>
}
}
}
@Html.ValidationMessageFor(m => Model.Value)
Sitecore Item Setup
In /sitecore/system/Settings/Forms/Field Types/Lists you will see several list types. This is where I added the new list type. I chose the Field Type template under /System/Forms/Field Type. I called it Country Drop Down List.
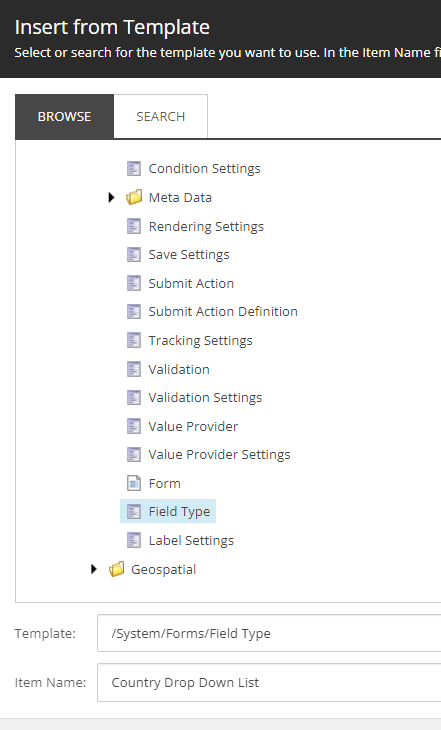
Under the settings I put the following. The rest I used the default.
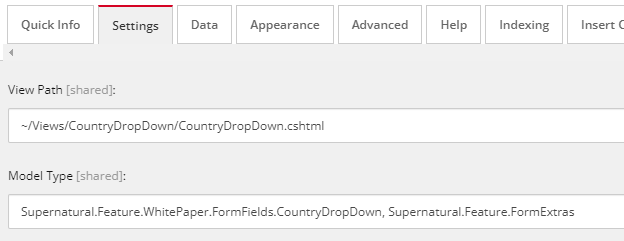
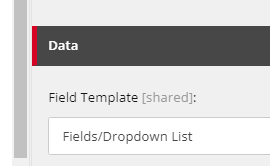
Under the appearance section. I chose Icons that match what the other lists had. Once important not is to make sure BackgroundColor was set to Grass to blend in with the other list field types.
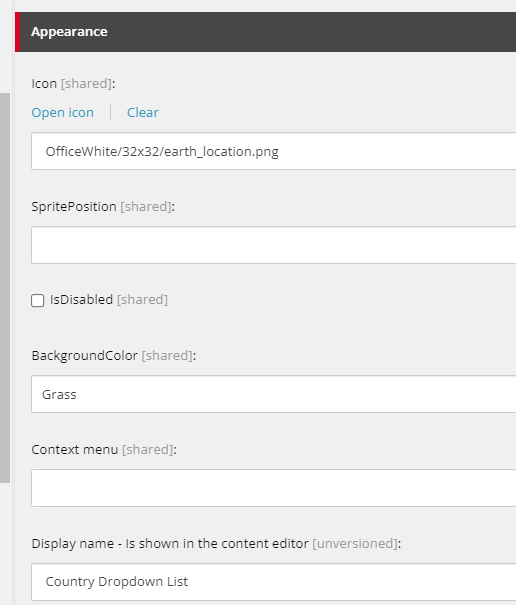
When you go to the Form Builder you will now see the Country Dropdown List as selection.
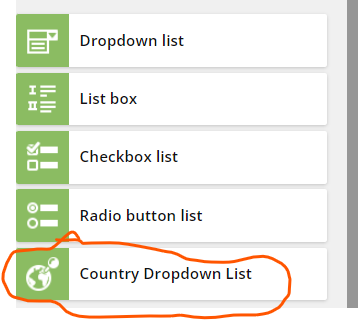
This is what the form will look like when the field is rendered. I clicked the down arrow in this example and US was the default.
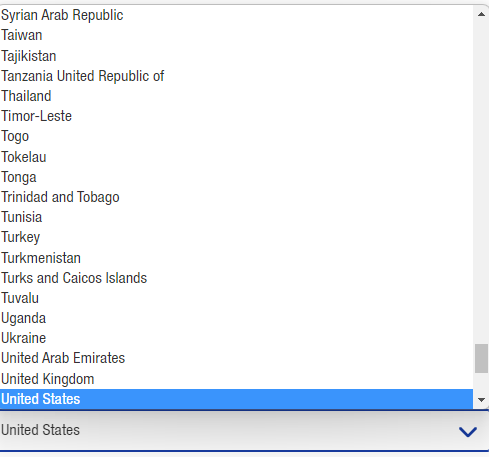
That is it. I may include this as a separate install in the near future. I will update this blog if I do.